Background
Cloudpress has a feature that allow users to export content from a Google Doc attached to a Trello card to their Content Management System like WordPress, Webflow, or other ones supported by Cloudpress. It is common in content writing teams to use Trello to manage their content calendar and track the progress of blog posts.
The specific feature in question allows a user to enable an option that will automatically export the content from an attached document whenever a card is moved to a specific column in Trello.
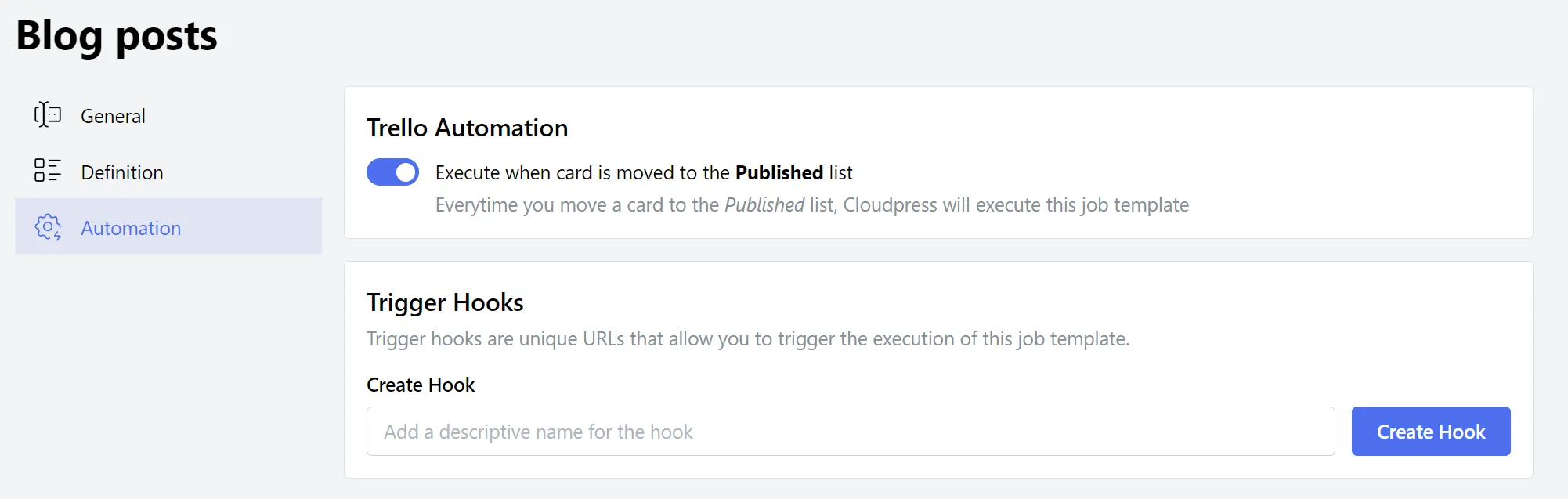
Whenever the user toggles the switch, Cloudpress will create or delete a webhook in Trello that will notify Cloudpress that the card was moved.
Sometimes it happens that the job that does the export is deleted in Cloudpress, but the underlying webhook in Trello is not deleted. This is not an issue as, per the Trello documentation, we can simply respond with and HTTP 410
status code, and that will delete the webhook in Trello.
I am using FastEndpoints in Cloudpress, so the high-level code in my endpoint ended up looking like the following:
public override async Task HandleAsync(RequestModel req, CancellationToken ct){ VerifySignature();
if (!WebhookIsValid(req)) { await SendAsync(null, StatusCodes.Status410Gone, ct); return; }
DoSomeWork();
await SendOkAsync(ct);}
Working around the ASP.NET Core status page
The issue I ran into was that the ASP.NET Core status code page middleware would intercept the response and redirect the user to the status page.
I used the UseStatusCodePagesWithReExecute
and this also ended up changing the status code from a status code 410
, resulting in Trello not deleting the webhook as it should.
app.UseStatusCodePagesWithReExecute("/Error/{0}");
I investigate this and came across this StackOverflow answer that suggested writing additional middleware to toggle the status code middleware for certain paths.
In my case, I was only interested in overriding the behaviour for one specific endpoint, so I handled this inside of the endpoint itself.
public override async Task HandleAsync(RequestModel req, CancellationToken ct){ var statusCodePagesFeature = HttpContext.Features.Get<IStatusCodePagesFeature>(); if (statusCodePagesFeature != null) { statusCodePagesFeature.Enabled = false; }
VerifySignature();
if (!WebhookIsValid(req)) { await SendAsync(null, StatusCodes.Status410Gone, ct); return; }
DoSomeWork();
await SendOkAsync(ct);}
The highlighted code above retrieves the status code page feature and then disable it. This means that, for this specific endpoint, the status code middleware is not executed and the correct HTTP status 410
is returned to Trello.